JiSoo's Devlog
[Vanila JS로 크롬 앱 만들기] Section 2 본문
JavaScript는 HTML을 읽어올 뿐만 아니라 변경도 가능

브라우저가 우리에게 많은 게 들어있는 document 객체를 준다
JavaScript로 정보를 가지고 올 수 있는 방법은 document 객체와 element를 가져오는 함수 사용하는 것
console.dir()은 element를 더 자세히 보여준다
const title = document.getElementById("title");
console.dir(title);
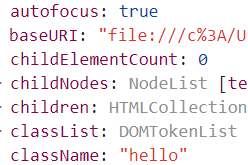
JavaScript는 HTML element를 가지고 오지만 HTML 자체를 보여주는 게 아니라 HTML을 표현하는 object를 보여준다
getElementById 함수를 통해 id로 element를 가져올 수 있고 변경도 가능하다
innerText, className, autofocus 등등
id보다는 보통 className을 사용하거나 둘 다 사용
많은 element 가져올 때 getElementByClassName 사용
tag name으로 element 가져오려면 getElementByTagName 사용
getElementByClassName, getElementByTagName은 배열을 가져오기 때문에 title.innerText와 같은 방법 사용 불가
<div class="hello">
<h1>Grab me!</h1>
</div>
querySelector는 element를 CSS 방식으로 검색 가능 → hello라는 class 내부에 있는 h1을 가지고 올 수 있다
const title = document.querySelector(".hello h1");
console.log(title);
const title = document.querySelector("div h1");
이렇게도 가능
querySelector는 단 하나의 element를 return
hello class 안에 많은 h1이 있다 해도 첫 번째 요소만 나온다
<div class="hello">
<h1>Grab me1!</h1>
</div>
<div class="hello">
<h1>Grab me2!</h1>
</div>
<div class="hello">
<h1>Grab me3!</h1>
</div>
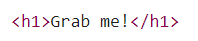
const title = document.querySelectorAll(".hello h1");
console.log(title);
querySelectorAll은 selector 안의 조건에 부합하는 모든 element를 가져다준다

id로 찾고 싶으면 querySelector("#hello") 이렇게 해주면 된다
getElementById("hello") == querySelctor("#hello")
const title = document.querySelector(".hello:first-child h1");
class hello를 가진 div 내부의 first-child인 h1을 찾아오는 것
const title = document.querySelector(".hello:first-child h1");
console.log(title);
title.style.color = "blue";
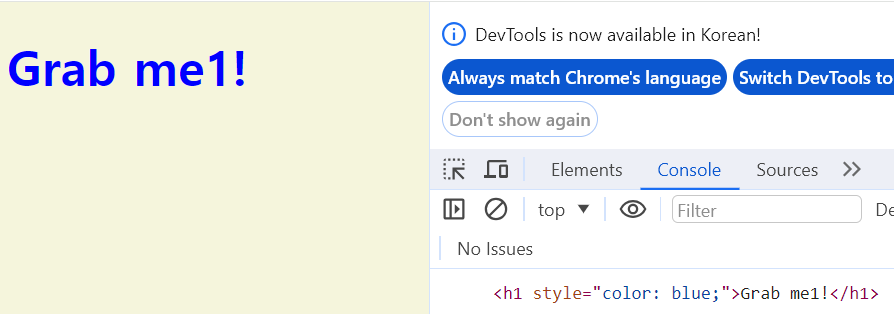
h1의 style을 JavaScript에서 변경 가능
JavaScript에서 대부분 작업할 일은 event를 listen 하는 것
event를 listen 하는 방법 ↓
HTML element를 가져와서 addEventListener를 호출하고 listen 하고 싶은 event 이름을 알려주고 event가 발생하면 호출할 함수 전달
const title = document.querySelector(".hello:first-child h1");
function handleTitleClick() {
console.log("title was clicked!");
}
title.addEventListener("click", handleTitleClick);
유저가 title을 click 할 경우에 JavaScript가 실행 버튼 대신 눌러주길 바라는 것
** event 발생 시 호출할 함수명 인자로 적을 때 () 적으면 안 된다

event는 on으로 시작하는 property이고 on 빼고 뒤에 거만 작성하면 된다 ex) onclick → click
const title = document.querySelector(".hello:first-child h1");
console.dir(title);
function handleTitleClick() {
title.style.color = "blue";
}
function handleMouseEnter() {
title.innerText = "Mouse is here!";
}
function handleMouseLeave() {
title.innerText = "Mouse is gone!";
}
title.addEventListener("click", handleTitleClick);
title.addEventListener("mouseenter", handleMouseEnter);
title.addEventListener("mouseleave", handleMouseLeave);
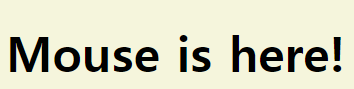

title.onClick = handleTitleClick;
title.onmouseenter = handleMouseEnter;
이렇게도 가능한데 addEventListener을 더 선호하는 이유는 removeEventListener를 통해 event listener를 제거할 수 있기 때문
window는 기본적으로 제공
document의 body, head, title 이런 것들은 중요하기 때문에 존재하고 나머지 element들은 querySelector나 getElementById 등으로 찾아와야 한다
function handleWindowResize() {
document.body.style.backgroundColor = "tomato";
}
window.addEventListener("resize", handleWindowResize);
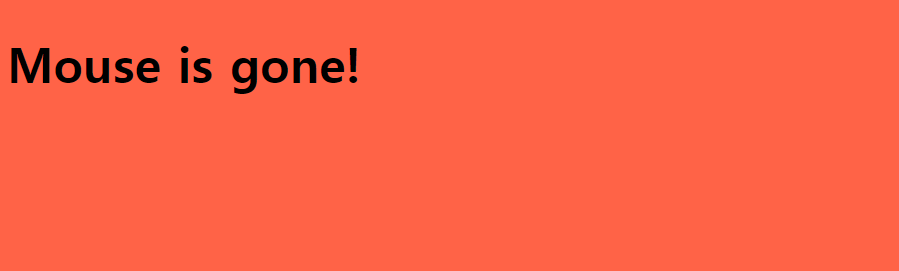
function handleWindowCopy() {
alert("copier!");
}
window.addEventListener("copy", handleWindowCopy);
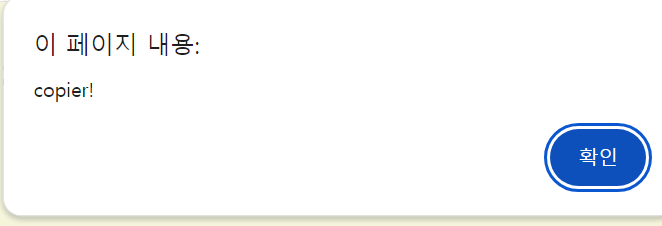
function handleWindowOffline() {
alert("SOS no WIFI");
}
function handleWindowOnline() {
alert("ALL GOOOD");
}
window.addEventListener("offline", handleWindowOffline);
window.addEventListener("online", handleWindowOnline);
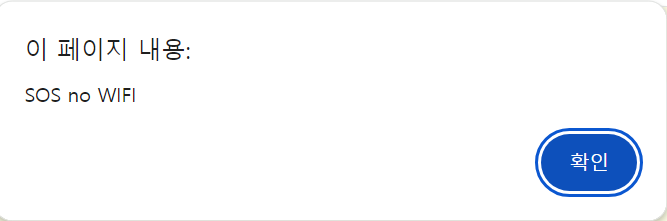
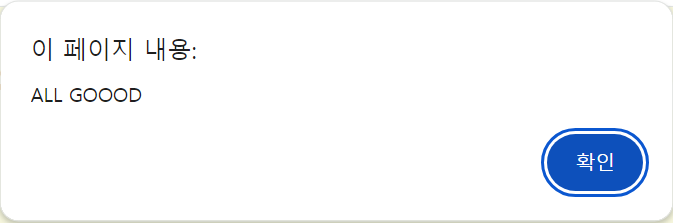
function handleTitleClick() {
if (h1.style.color === "blue") {
h1.style.color = "tomato";
} else {
h1.style.color = "blue";
}
}
h1.addEventListener("click", handleTitleClick);
이렇게 할 수도 있지만 color의 현재 상태를 저장해서
function handleTitleClick() {
const currentColor = h1.style.color;
let newColor;
if (currentColor === "blue") {
newColor = "tomato";
} else {
newColor = "blue";
}
h1.style.color = newColor;
}
element 찾기 → event를 listen → 그 event에 반응
h1 {
color: cornflowerblue;
transition: color 0.5s ease-in-out;
}
.active {
color: tomato;
}
↑ CSS
function handleTitleClick() {
if (h1.className === "active") {
h1.className = "";
} else {
h1.className = "active";
}
}
active는 그저 class name 일뿐
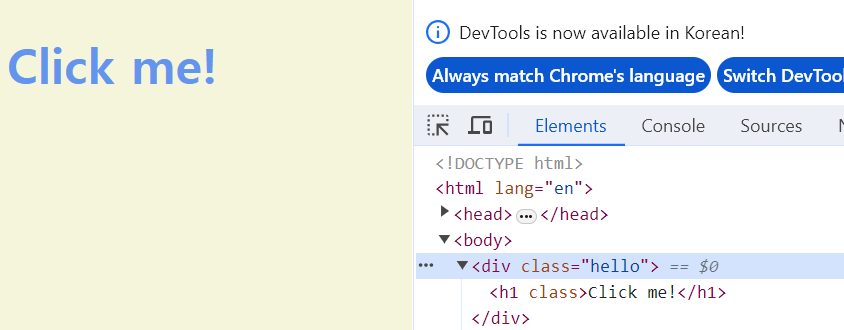

function handleTitleClick() {
const clickedClass = "clicked";
if (h1.className === clickedClass) {
h1.className = "";
} else {
h1.className = clickedClass;
}
}
이렇게 string을 변수에 저장하는 게 유용
class name을 바꾸는 다른 방법 → classList 사용
classList는 class들을 목록으로 작업할 수 있게 허용
function handleTitleClick() {
const clickedClass = "clicked";
if (h1.classList.contains(clickedClass)) {
h1.classList.remove(clickedClass);
} else {
h1.classList.add(clickedClass);
}
}
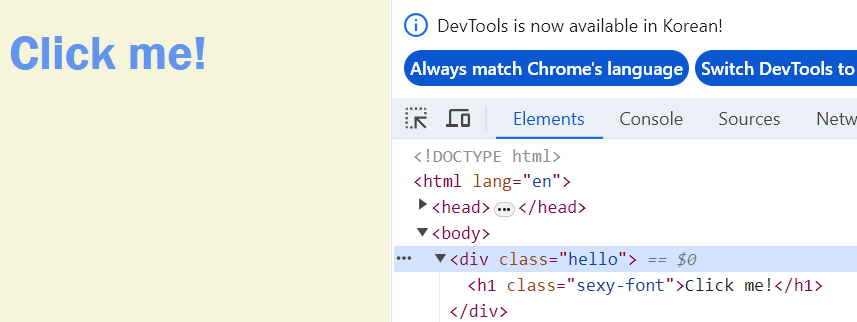
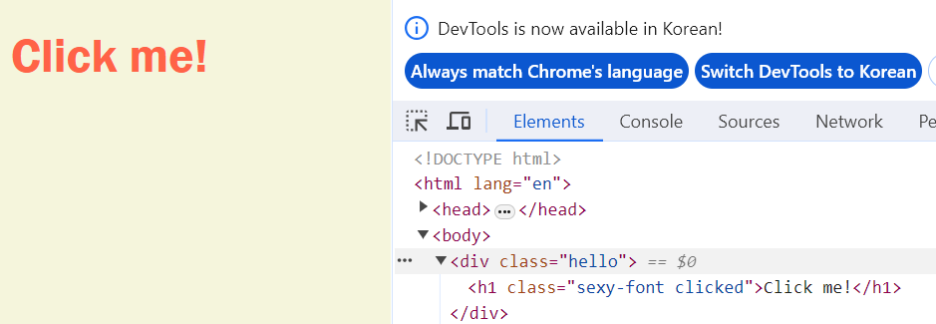
toggle function은 class name이 존재하는지 확인
function handleTitleClick() {
h1.classList.toggle("clicked");
}
toggle은 h1의 classList에 clicked class가 이미 있는지 확인해서 있으면 toggle이 clicked 제거, 없으면 clicked를 classList에 추가
toggle은 토큰이 존재하면 토큰 제거, 존재하지 않는다면 토큰 추가
'Frontend > JS' 카테고리의 다른 글
[Vanila JS로 크롬 앱 만들기] Section 5 (0) | 2024.01.03 |
---|---|
[Vanila JS로 크롬 앱 만들기] Section 4 (0) | 2024.01.03 |
[Vanila JS로 크롬 앱 만들기] Section 3 (1) | 2024.01.03 |
[Vanila JS로 크롬 앱 만들기] Section 1 (2) | 2024.01.03 |