JiSoo's Devlog
[Vanila JS로 크롬 앱 만들기] Section 5 본문
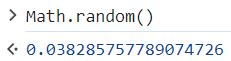
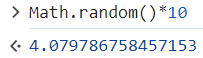
Math.random()에 10을 곱하면 0에서 10 사이의 숫자 얻을 수 있다
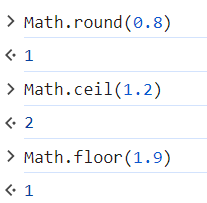
floor 내림
ceil 올림

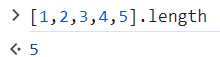
const todaysQuote = quotes[Math.floor(Math.random() * quotes.length)];
quote.innerText = todaysQuote.quote;
author.innerText = todaysQuote.author;

<img src="img/0.jpeg"/>
이렇게 html로 만들어야 하는 것을
const images = ["0.jpg", "1.jpg", "2.jpg"];
const chosenImage = images[Math.floor(Math.random() * images.length)];
const bgImage = document.createElement("img");
console.log(bgImage);
bgImage.src = `img/${chosenImage}`;
createElement(" ")는 JS에서 html 요소 생성
예시에서는 html 내에 img 태그 생성
document.body.appendChild(bgImage);
appendChild()는 body에 html을 추가할 것이다
document.body.prepend(bgImage);
prepend()도 사용 가능한데 append는 body에서 가장 뒤에, prepend는 가장 위에 오게

item이 5개 있는 Array에서 마지막 item을 가져오려면 필요한 숫자는 4
<form id="todo-form">
<input type="text" placeholder="Write a To Do and Press Enter" required />
</form>
<ul id="todo-list"></ul>
const toDoForm = document.getElementById("todo-form");
const toDoInput = document.querySelector("#todo-form input");
const toDoList = document.getElementById("todo-list");
function handleToDoSubmit(event) {
event.preventDefault();
const newTodo = toDoInput.value;
toDoInput.value = "";
}
toDoForm.addEventListener("submit", handleToDoSubmit);
const newTodo = toDoInput.value 이 문장에서 하는 건 input의 현재 value를 새로운 변수에 복사하는 것
그다음에 무엇을 하든, newToDo 변수와는 아무 상관 X
newTodo는 input의 value를 비우기 전의 값
const li = document.createElement("li");
const span = document.createElement("span");
li.appendChild(span);
}
function handleToDoSubmit(event) {
event.preventDefault();
const newTodo = toDoInput.value;
toDoInput.value = "";
paintTodo(newTodo);
}
li.appendChild를 통해 li가 span이라는 자식을 가지게 된다
function paintTodo(newTodo) {
const li = document.createElement("li");
const span = document.createElement("span");
li.appendChild(span);
span.innerText = newTodo;
toDoList.appendChild(li);
}
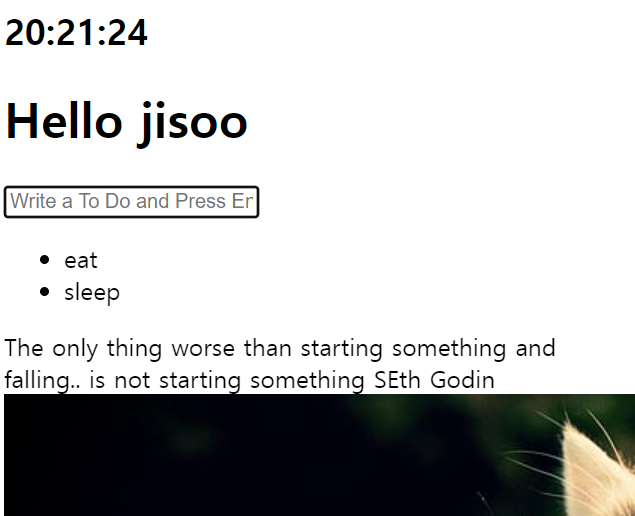
function deleteToDo(event) {
const li = event.target.parentElement;
li.remove();
}
function paintTodo(newTodo) {
const li = document.createElement("li");
const span = document.createElement("span");
span.innerText = newTodo;
const button = document.createElement("button");
button.innerText = "❌";
button.addEventListener("click", deleteToDo);
li.appendChild(span);
li.appendChild(button);
toDoList.appendChild(li);
}
target은 button, button은 부모를 갖고 있고 그 부모인 li에 접근해 제거
const toDos = [];
function saveToDos() {
localStorage.setItem("todos", toDos);
}
toDos array를 localStorage에 집어넣기
function saveToDos() {
localStorage.setItem("todos", JSON.stringify(toDos));
}
array 모양으로 저장
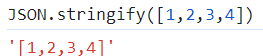
값을 단순한 string으로 변경
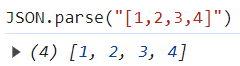
JavaScript가 이해할 수 있는 살아있는 array로 만들 수 있다
string 데이터 타입을 object로 바꾼 건데 이 object는 array 같이 바뀐다
function sayHello(item) {
console.log("this is the turn of", item);
}
const savedToDos = localStorage.getItem(TODOS_KEY);
if (saveToDos !== null) {
const parsedToDos = JSON.parse(savedToDos);
parsedToDos.forEach(sayHello);
}
forEach는 array의 각 item에 대해 function을 실행하게 해준다
if (saveToDos !== null) {
const parsedToDos = JSON.parse(savedToDos);
parsedToDos.forEach((item) => console.log("this is the turn of", item));
}
이 함수를 호출하면서 array에 있는 각각의 item을 준다
이전에 입력한 todo와 새로 입력한 todo 모두 유지하고 싶다면
let toDos = [];
const를 let으로 바꿔 업데이트 가능하도록
if (savedToDos !== null) {
const parsedToDos = JSON.parse(savedToDos);
toDos = parsedToDos;
parsedToDos.forEach(paintTodo);
}
forEach 함수는 paintToDo를 parsedToDos 배열의 요소마다 실행
forEach는 paintToDo를 기본적으로 실행
function ExFilter(){
}
[1, 2, 3, 4].filter(ExFilter);
filter는 ExFilter에 1, 2, 3, 4를 넣어 부를 것이다
ExFilter 함수는 만약 새 array에도 1, 2, 3, 4를 포함하고 싶으면 반드시 true를 리턴해야 한다
false를 리턴하면 그 item은 새 array에 포함되지 않을 것이다
navigator.geolocation.getCurrentPosition()
getCurrentPosition은 인자 2개가 필요한데 모든 게 잘 됐을 때 실행될 함수, 에러 발생했을 때 실행될 함수
function onGeoOk(position) {
const lat = position.coords.latitude;
const lng = position.coords.longitude;
console.log("You live in", lat, lng);
}
function onGeoError() {
alert("Can't find you. No weather for you");
}
navigator.geolocation.getCurrentPosition(onGeoOk, onGeoError);

user의 위치 파악 가능
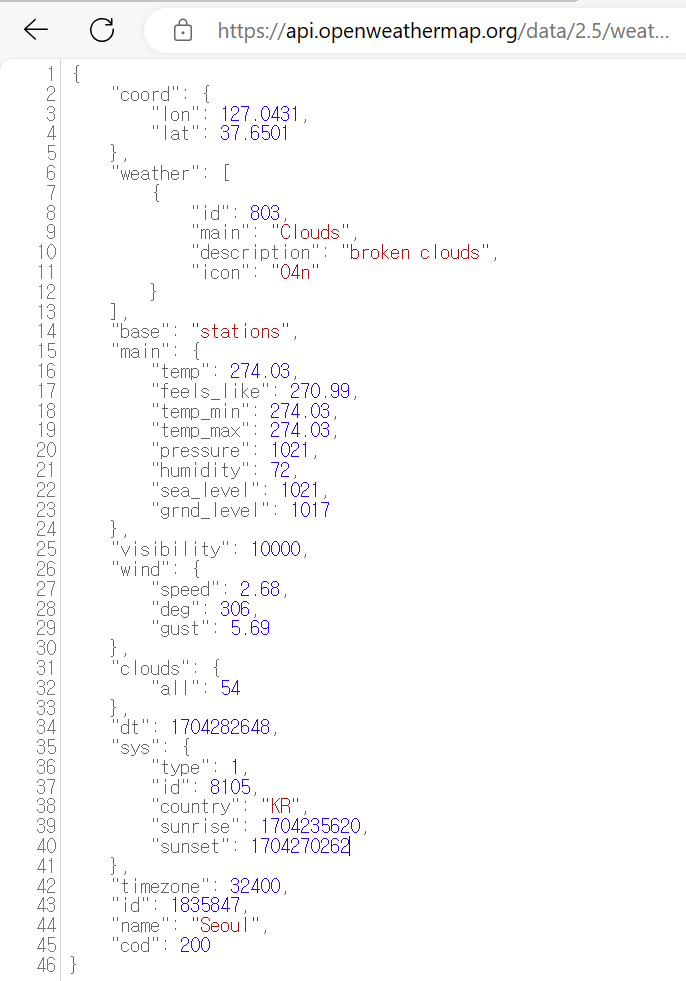
latitude, longitude, API Key와 함께 API 호출
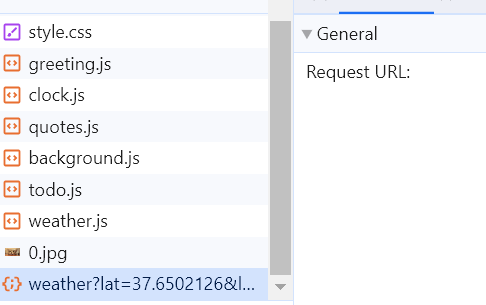
function onGeoOk(position) {
const lat = position.coords.latitude;
const lon = position.coords.longitude;
const url = `https://api.openweathermap.org/data/2.5/weather?lat=${lat}&lon=${lon}&appid=${API_KEY}&units=metric`;
fetch(url);
}
fetch(url)
.then((response) => response.json())
.then((data) => {
console.log(data.name, data.weather[0].main);
});
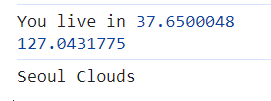
fetch(url)
.then((response) => response.json())
.then((data) => {
const weather = document.querySelector("#weather span:first-child");
const city = document.querySelector("#weather span:last-child");
city.innerText = data.name;
weather.innerText = `${data.weather[0].main} / ${data.main.temp}`;
});

'Frontend > JS' 카테고리의 다른 글
[Vanila JS로 크롬 앱 만들기] Section 4 (0) | 2024.01.03 |
---|---|
[Vanila JS로 크롬 앱 만들기] Section 3 (1) | 2024.01.03 |
[Vanila JS로 크롬 앱 만들기] Section 2 (0) | 2024.01.03 |
[Vanila JS로 크롬 앱 만들기] Section 1 (2) | 2024.01.03 |